Minting $1,500,000 at the Federal Reserve of Foo Fighters
A little over a week ago, the Foo Fighters played both Reading and Leeds festivals in the UK. Each of these shows ended in a confetti cannon launch of several hundred thousand fake FOO $100 bills. On the backside of each of these bills we subtly scrawled the web address inrockwetrust.co.uk. I was tasked with building an experience that carried this theme onto the web at that URL.
What made this project really fun was how seamlessly we found ourselves (and our fans) going back and forth from real life to the web and it’s a perfect example of just how connected these two spaces can be. So before we talk about the Internet, let’s take a look at what went into the real world event, as it was literally launched into fans' faces.
Is this the Real Life?
Master Die
The original bill was designed by Jason and Doug over at Morning Breath whom you may know from Wasting Light, Era Vulgaris, and Slayer. The final version is fucking rad and management was able to add a ton of hidden messages and band references in addition to the inrockwetrust.co.uk URL.
On a personal note, I just hired Morning Breath to design something for me, but you’ll have to wait and see what that’s all about.
Raining Money
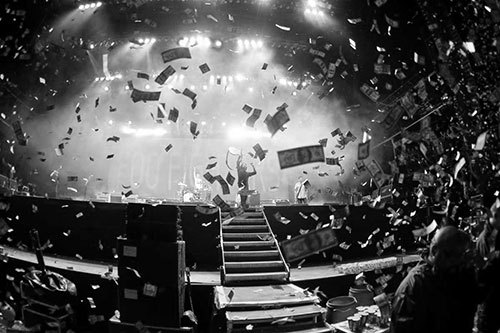
Foo Fighters coordinated with the SFX geniuses over at Quantum Special Effects to turn the confetti launch into a reality, employing their entire arsenal of 24 stadium shot cannons. Then on August 24th, at the end of the first set and despite rain, the band launched a shit ton of fake Foo Fighters dollar bills.
Fans in the front were lucky enough to grab some and photos like this started trickling onto the web in no time. It didn’t take them long to decipher the secret URL on the back and head over to inrockwetrust.co.uk.
But what was waiting for them when they got there?
Is this just Internet?
Having just come off the Bloc Party Four campaign, where I did a lot of server side image manipulation, I thought it would be a good idea to allow fans to strike their best presidential pose and become part of the FOO bill. Initially I setup an app similar to Four, in which your Facebook profile pic was pulled in automatically and placed inside the bill. This automation made it difficult to frame the face appropriately, and forced me to make two very important decisions:
- Allow users to utilize their webcam to frame themselves within the bill
- Allow users to participate anonymously (no Facebook login)
Anonymous? Don’t you want those emails, dude?
My thinking was that we were already adding a barrier of entry by requiring a webcam, and removing any login requirements should make up for this.
Ok, but what did the band get out of this?
Look, you don’t always have to sell something. Try being cool for the sake of being cool every now and then. You’d be surprised how well that works out in the long run.
Now then, let’s talk programming.
Webcam Portrait Gallery
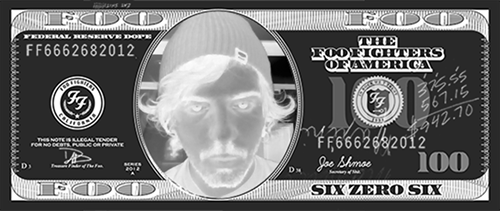
Utilizing a user’s webcam is pretty straightforward these days, but talking to different webcam types within different environments while also using the best available technology can be a little tricky. That’s where getUserMedia.js comes in. Written by Addy Osmani, this library exists as a shim that calls on WebRTC for Chrome, Opera 12, and Firefox nightlies. All other environments fallback to the also excellent flash powered jQuery-webcam plugin, written by Robert Eisele.
Rather than explaining that setup here, I’d suggest checking out Addy’s well documented demo app. I would, however, like to share how I was able to get the webcam image over to my server (from both webrtc and flash) for manipulation as that was a bit of a learning experience. Both methods consist of putting the captured image onto a temporary canvas and getting a Base64 representation of that image which is then sent over to the server.
For WebRTC, it couldn’t be easier:
// Get the HTML5 video element
video = document.getElementsByTagName('video')[0];
// Draw that element onto the canvas
App.context.drawImage(video, 0, 0, App.options.width, App.options.height);
// Use toDataURL to get your Base64 representation
App.canvas.toDataURL('image/png');
Flash, that son of a bitch, makes it a little harder for us:
// Split up the image data into columns
col = data.split(;);
// Declare some variables, img comes from a getImageData() called in `init`
img = App.image;
w = 320;
h = 240;
// Loop through each of the image columns and place into canvas image
for (i = 0; i <= 319; i += 1) {
tmp = parseInt(col[i]);
img.data[App.pos + 0] = (tmp >> 16) & 0xff;
img.data[App.pos + 1] = (tmp >> 8) & 0xff;
img.data[App.pos + 2] = tmp & 0xff;
img.data[App.pos + 3] = 0xff;
App.pos += 4;
}
// Once completed, you can put it onto our temporary canvas and use toDataURL
if (App.pos >= 4 * w * h) {
App.context.putImageData(img, 0, 0);
App.canvas.toDataURL('image/png');
}
On the server side I’m running a very basic Sinatra app that accepts the image, temporarily stores it as a StringIO, and saves it to my S3 using Carrierwave.
image = params[:image].gsub("data:image/png;base64,", "")
io = FilelessIO.new(Base64.decode64(image))
io.original_filename = "bill.png"
io.content_type = "image/png"
bill = Bill.create(name: params[:name])
bill.image = io
bill.save
In doing so the image passes through all of my Carrierwave processors and gets minted accordingly:
Minting the Money
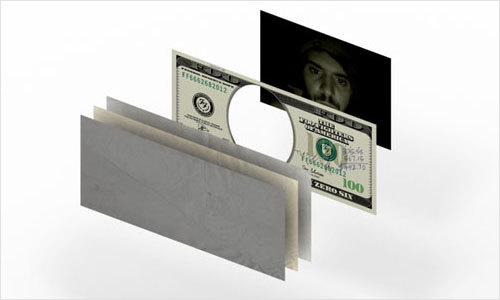
The first step in minting is to use MiniMagick to turn the full color webcam photo grayscale and then give it a nice money green tint.
img.combine_options do |c|
c.colorspace 'Gray'
c.fill '#dfdfc8'
c.tint '100'
end
Morning Breath was nice enough to provide me with the layered Photoshop source file for the bill, which I used as a guide for setting up my composition functions. In addition to the bill image, three different textures were overlaid on top using the Multiply composition method. This allowed the textures to bleed through and leave a nice big crease across the users face.
img = img.composite(texture) do |c|
c.compose "Multiply"
c.gravity "NorthWest"
end
One thing you’ll notice on the front of the original bill is the name “Claire” is written in pen. Now I won’t tell you who that is, but I will tell you how I mimicked this on my version, allowing the user to provide their own name and writing it on the bill.
First, we setup a nice handwritten typeface by passing in the TTF file path manually. Then we give the type a nice pen color and size it up for writing. Finally, we draw the provided name over the user’s face.
img.combine_options do |c|
c.gravity 'NorthWest'
c.font 'public/learning.ttf'
c.fill "rgb(73,70,130)"
c.pointsize '60'
c.draw "text 135,70 '#{ model.name }'"
end
Raining Money II
While it isn’t as exciting as shooting off 24 stadium cannons… the CSS3 animation used to mimic a falling bill is actually quite awesome, and the live feed of data had the benefit of showing me errors… literally falling in front my face. Anyway… all it took was a read through of this guide and a few keyframe animations:
/* Fall from the top to bottom */
@keyframes fall {
0% { top: -200px; }
100% { top: 1000px; }
}
/* Flip and rotate the front */
@keyframes front {
from { transform: rotate(-50deg) rotateX(0deg); }
to { transform: rotate(50deg) rotateX(360deg); }
}
/* Flip and rotate the back */
@keyframes back {
from { transform: rotate(-50deg) rotateX(-180deg); }
to { transform: rotate(50deg) rotateX(180deg); }
}
Is this the Real Life?
What? That’s not how that Queen song goes.
I know.
However, there was one more idea that didn’t make it into the final campaign that brought us back to real life once again. You see, I wrote a piece of code that would actually print the bills on a printer I controlled as they were being generated by users. In the end, it seemed like a big waste of paper and would quickly get out of control given the velocity of minted bills.
I plan on revisiting this technique in a future blog post about building your own Instagram printer. If you’re interested in that, I’d suggest subscribing to my newsletter.
As always, thanks for reading, and please let me know if you have any questions or comments via Email or Twitter.